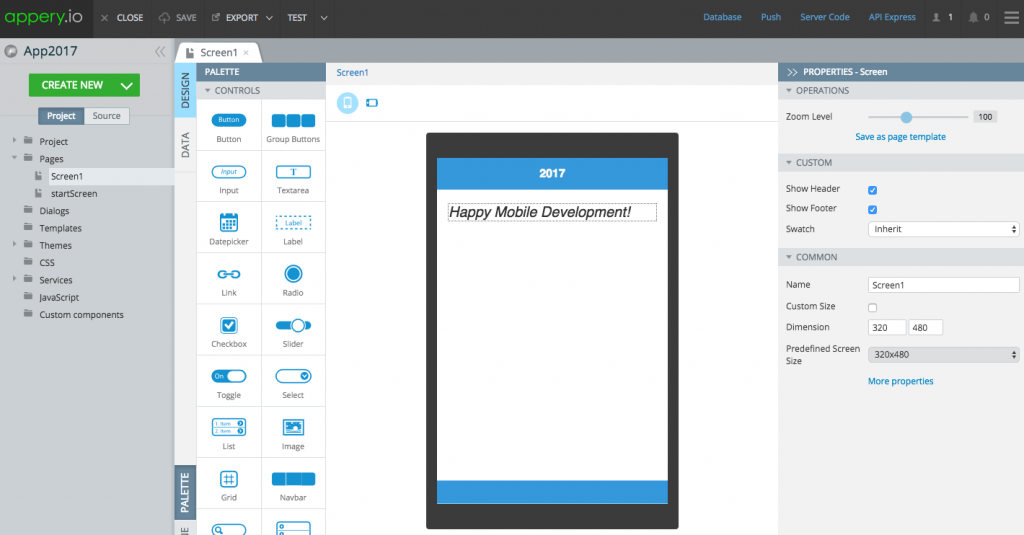
Happy New Year!
As we are starting 2017, we want to let you know about all the Appery.io resources available to you, to help you build you app faster and easier. These resources are being updated with new content all the time, so we definitely recommend subscribing to updates or following them. This way you will be always up to date with what is happening. Let’s get to the resources.
Developer Documentation and Portal (http://docs.appery.io)
The docs site is the most important resources (obviously). The docs site contains the platform documentation, tutorials, and API reference section. We regularly update the content on this site. If you find a typo or an error, please use the Suggest Edits feature to tell us about it.
The docs site has a blog section (http://docs.appery.io/blog) where we usually post technical information such as API updates or library updates. The information is usually very technical and specific so it doesn’t go on the main blog. We will also post links to tutorials and videos.
The Appery.io blog (http://blog.appery.io)
The blog is one of the most important resources you should read. This is where we post platform updates, announcements, new videos, new tutorials, case studies and anything else. We definitely recommend you follow the blog to stay up to date. Following the blog is very simple. You can subscribe via the RSS feed or subscribe via email (you will get an email every time a new post is published).
YouTube Channel (http://youtube.com/apperyio)
This is our most popular resource. Our YouTube channel has over 200 short videos on various topics to help you build apps faster. Definitely subscribe to get updates when we publish new videos. The videos are organized into playlists:
Most of our videos are no longer than 15 minutes. This allows you to learn about a topic, feature or benefit very fast and you won’t be bored through an hour long example.
Community Forum (http://appery.io/forum)
Our community forum is the place to get help, ask questions and help your fellow Appery.io developers.
Platform Status Page (http://status.appery.io)
Appery.io platform status page shows each platform component and its current status. It’s important to bookmark or follow this page via RSS to always know the status of each component.
Twitter (http://twitter.com/apperyio)
Twitter is where we post important announcements, platform updates, new tutorials, case studies, new videos and anything else. We recommend to follow up on Twitter to always stay up to date.
Facebook Page (http://facebook.com/apperyio)
Facebook is very similar to Twitter. On Facebook we post important announcements, platform updates, new tutorials, case studies, and new videos. We recommend to Like us Facebook to always stay up to date.
LinkedIn Company Page (https://www.linkedin.com/company/appery-io)
On our LinkedIn Company Page we post announcements, new tutorials, case studies, and new videos.
Google+ Page (https://plus.google.com/u/0/104276681162289155352)
Yes, we are still on Google+ :).
Instagram (https://www.instagram.com/apperyio/)
This is a new resource and we will be posting a lot more in 2017.
What’s New Panel
And a bonus resource is our What’s New panel. The What’s New panel can be opened from any platform page from the header. The panel shows you the most recent updates, tutorials, and videos. Check this resource often to learn what’s new.
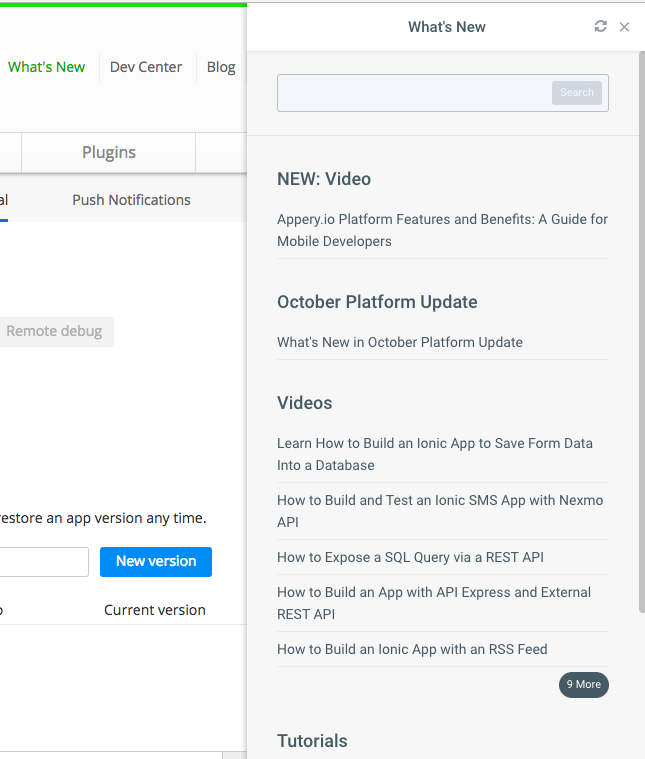