How to Perform Basic Database Operations: Create, Read, Update and Delete
The Appery.io Backend Services consists of the following components:
- Database – for storing any app data.
- Server Code – for writing server-side app logic using JavaScript.
- API Express – integrating with external systems and APIs.
- Push Notifications – sending push messages to devices.
- Web Hosting – publishing and hosting mobile web apps.
In this post, we will show you how a Server Code script integrates with the Database.
One of the most common questions we get is how to work with the database, how to create, edit and delete data. This makes sense as virtually any mobile app needs to store data in a database and perform these basic operations. To access the database, we are going to use Server Code script. Server Code allows writing app logic using JavaScript which will be executed on the server. For example, a script can access the database, send a Push Notification message, sort data and invoke an external REST API. We will start with the most basic operation: how to read data from the database.
The database collection used in this post looks like this:

Database collection.
This can be data collected from a form inside an app – a form that collects information about the user.
Reading Data
Server Code script has out-of-the-box API to access the database to perform all the basic operations. The following script reads all objects from the above collection.
var dbApiKey = "fe7c124b-f7c5-4764-9274-173b56a97102"; var result = Collection.query(dbApiKey, "Data"); Apperyio.response.success(result, "application/json");
One line 2, the script retrieves all the data.
One line 3, the script response is set. A Server Code script is invoked as a REST API. The code on this line defines the API response.
A script can be quickly tested from the Run tab where you can see the JSON data:
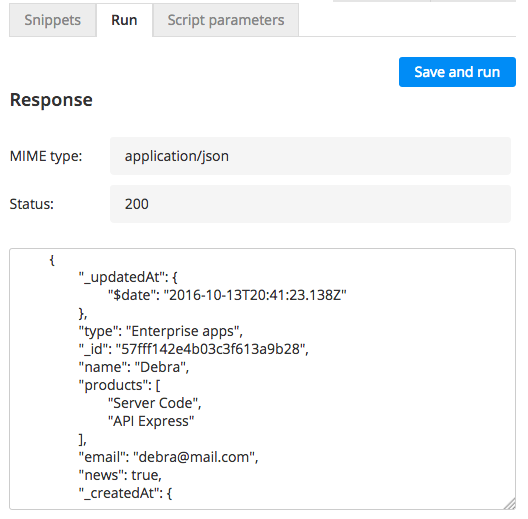
Testing the script.
Reading data from a database collection is fast and simple. Next, we are going to save data into the database.
Creating Data
The following script saves a new record into the database collection:
var dbApiKey = "fe7c124b-f7c5-4764-9274-173b56a97102"; var result = Collection.createObject(dbApiKey, "Data", { "name": "Tina", "email": "tina@appery.io", "news": true, "products": ["API Express", "Server Code"], "type": "Consumer apps" }); Apperyio.response.success(result, "application/json");
The script specifies all the fields (columns). It’s not required to specify all the fields. If some fields are not included then those fields will be empty in the database collection.
A response from a create operation is the object ID and the time when the object was created:
{ "_id": "57fffdd0e4b0cd86af6a31e6", "_createdAt": { "$date": "2016-10-13T21:34:08.377Z" } }
Creating a new record is also simple. Next, we are going to show a script which updates an existing record.
Updating Data
To update a record you need to know the record ID. The update operation is similar to the create operation. You can also specify only the fields that need updating. For example, using the record from Creating Data step, the following script updates the email and news fields:
var dbApiKey = "fe7c124b-f7c5-4764-9274-173b56a97102"; var result = Collection.updateObject(dbApiKey, "Data", "57fffdd0e4b0cd86af6a31e6", { "email": "tina.dylan@appery.io", "news": false });
The extra parameter in the update operation is the object ID.
The response from an update operation is the timestamp of the update.
Deleting Data
To delete a record we only need to know the record ID. The following script deletes a record from the database collection:
var dbApiKey = "fe7c124b-f7c5-4764-9274-173b56a97102"; var result = Collection.deleteObject(dbApiKey, "Data", "57fffdd0e4b0cd86af6a31e6");
The delete operation doesn’t return any response besides a 200 HTTP response code.
Why Use Server Code to Access the Database?
You might be wondering why not access the database directly using its REST API instead of using Server Code. We recommend using Server Code to access the database because most apps will require additional logic around database access. For example, if you need to sort or filter the data before displaying in the app, it’s best to do it on the server. You might want to validate data before saving it into the database. Also, you might want to run additional logic before or after the operation. For example, you can invoke an external REST API or send an email or send an SMS message or a Push Notification. All this easily done using Server Code but would be a lot more challenging if the app accessed the database directly.
Summary and Resources
Performing the basic database operations is simple using the Server Code. To make development faster, we recommend using the Snippets panel to quickly insert most commonly used code into the script. The examples in this blog post were created entirely using the Snippets.
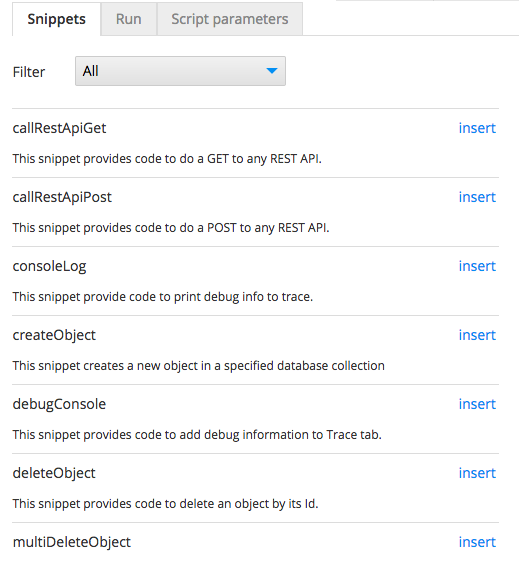
Server Code Snippets
We have many more resources to help building mobile apps: